Class 12 Informatics Practices Worksheet: DataFrame Attributes
Part A: Fill in the Blanks
- The attribute used to get the number of rows in a DataFrame is __________.
- To get the column names of a DataFrame, use the __________ attribute.
- The __________ attribute returns the index of a DataFrame.
- To access the data types of each column in a DataFrame, use the __________ attribute.
- The attribute that returns the number of elements in a DataFrame is __________.
Part B: True or False
- The
df.shape
attribute returns a tuple representing the number of rows and columns. (True / False) - The
df.columns
attribute provides the list of row indices in a DataFrame. (True / False) - The
df.index
attribute provides the index labels of a DataFrame. (True / False) - The
df.dtypes
attribute gives the data types of the DataFrame’s columns. (True / False) - The
df.size
attribute returns the total number of elements in the DataFrame. (True / False)
Part C: Short Answer Questions
- What does the
df.shape
attribute represent? Provide an example. - How can you retrieve the names of all columns in a DataFrame using attributes? Write the code.
- Explain the difference between
df.index
anddf.columns
. - How can you find out the data type of each column in a DataFrame? Provide an example.
- Write the code to get the total number of elements in a DataFrame named
df
.
Part D: Practical Exercises
Given the following DataFrame:
import pandas as pd data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [24, 27, 22], 'City': ['New York', 'Los Angeles', 'Chicago'] } df = pd.DataFrame(data)
Perform the following tasks:
- Print the number of rows and columns using
df.shape
. - Retrieve and print the column names using
df.columns
. - Get and print the index labels using
df.index
. - Find and print the data types of each column using
df.dtypes
. - Determine and print the total number of elements using
df.size
.
- Print the number of rows and columns using
Create a DataFrame with the following data:
Product Price Stock A 100 50 B 150 30 C 200 20 Write the code to:
- Access and print the
columns
attribute. - Access and print the
index
attribute. - Access and print the
dtypes
attribute. - Print the
shape
attribute.
- Access and print the
Given the DataFrame
df
:import pandas as pd data = { 'Employee': ['John', 'Jane', 'Tom', 'Lucy'], 'Salary': [50000, 55000, 60000, 65000], 'Department': ['HR', 'Finance', 'IT', 'Admin'] } df = pd.DataFrame(data)
Write the code to:
- Print the number of rows and columns.
- Print the data type of the
Salary
column. - Print the total number of elements in the DataFrame.
Part E: Code Correction
Examine the following code snippets and correct any mistakes related to DataFrame attributes. Rewrite the correct code.
import pandas as pd data = { 'Name': ['Anna', 'Ben', 'Charlie'], 'Age': [22, 23, 25] } df = pd.DataFrame(data) print(df.shape) # Correct print(df.columns) # Correct print(df.index()) # Incorrect
import pandas as pd data = { 'Product': ['X', 'Y', 'Z'], 'Price': [10, 20, 30] } df = pd.DataFrame(data) print(df.dtypes) # Correct print(df.size) # Correct print(df.columns()) # Incorrect
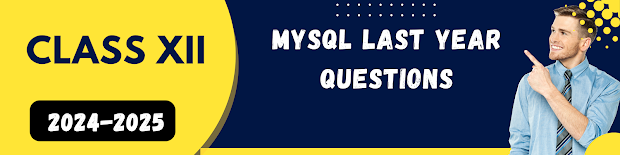.png)
1 Comments
HELLO SIR
ReplyDeletePlease do note create link post in comment section