Data Visualization is the graphical representation of data to help users understand patterns, trends, and insights. Visualization tools like Matplotlib are commonly used to transform raw data into visual forms, which makes analysis more accessible and meaningful.
Purpose of Plotting
Plotting data has several key purposes:
- Simplifies Data Understanding: Visuals make data easier to interpret than raw numbers.
- Reveals Patterns and Trends: Allows us to spot trends, outliers, and relationships in the data.
- Enables Better Decision-Making: Data-driven decisions are easier to make with clear, visualized insights.
- Communicates Information Effectively: Plots can simplify complex data, making it easier to present findings to others.
Common Types of Plots Using Matplotlib
Matplotlib is a Python library used to create a variety of static, animated, and interactive plots.
1. Line Plot
A line plot is used to display data points connected by a continuous line. It is ideal for visualizing trends over time or a sequence.
Code Example:
Saving the Plot:
2. Bar Graph
A bar graph displays data using rectangular bars, ideal for comparing quantities across categories.
Code Example:
3. Histogram
A histogram shows the distribution of data by grouping values into bins and plotting the frequency of values in each bin. It’s ideal for understanding data distribution.
Code Example:
In Python, with libraries like Matplotlib, you can customize plots by adding labels, titles, and legends to make them more informative. Here’s a quick guide on how to do it.
1. Adding Labels to the Axes
Use plt.xlabel()
and plt.ylabel()
to label the x and y axes:
2. Adding a Title
Use plt.title()
to add a title to your plot:
3. Adding a Legend
Use plt.legend()
to add a legend, which is especially useful when you have multiple lines or datasets in a single plot. You can either set the label within the plot
function or pass it to legend()
.
Complete Example
Here’s a complete example putting it all together:
Adding Labels to a Plot
To add labels to the x-axis and y-axis in a Matplotlib plot, you can use plt.xlabel()
and plt.ylabel()
. Here’s a basic example that demonstrates how to add these labels to a plot.
Example: Adding Labels to a Plot
Explanation
plt.xlabel('Time (s)')
: Sets the label for the x-axis, here labeled as “Time (s)”.plt.ylabel('Distance (m)')
: Sets the label for the y-axis, here labeled as “Distance (m)”.plt.title('Distance vs Time')
: Adds a title to the plot, here titled as “Distance vs Time”.
This customization makes the plot clearer, especially in conveying what each axis represents. You can replace "Time (s)"
and "Distance (m)"
with any other descriptive text that fits your data.
output
Adding a Title to a Plot
To add a title to a plot in Matplotlib, you can use the plt.title()
function. This helps provide context for what the plot represents.
Example: Adding a Title to a Plot
Explanation
plt.title('Sales Growth Over Time')
: This adds a title to the plot, here titled as “Sales Growth Over Time.”
Adding a Legend to a Plot
In Matplotlib, a legend can be added to clarify the meaning of multiple lines or datasets in a plot. Use the plt.legend()
function to add this, which will display labels defined in label
attributes within the plot.
Example: Adding a Legend to a Plot
Explanation
label='Product A'
andlabel='Product B'
: These labels are assigned to each plot, defining what each line represents.plt.legend()
: This command displays the legend box, which shows the labels forProduct A
andProduct B
with their respective line styles.
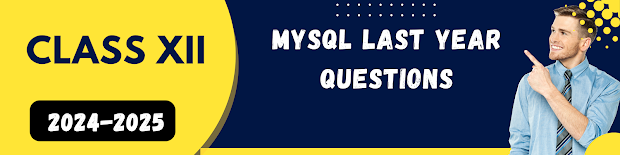.png)
0 Comments
Please do note create link post in comment section