Worksheet: Dropping Rows and Columns in DataFrames
Section A: Multiple Choice Questions (MCQs)
Which method is used to drop rows or columns from a DataFrame?
- a)
delete()
- b)
remove()
- c)
drop()
- d)
pop()
- a)
What parameter is used with the
drop()
method to specify whether you are dropping rows or columns?- a)
axis
- b)
index
- c)
level
- d)
key
- a)
If you want to drop a row with label 'A', what would be the correct way to do this?
- a)
df.drop(columns='A')
- b)
df.drop(index='A')
- c)
df.drop(row='A')
- d)
df.drop(axis='A')
- a)
What does
inplace=True
do when used with thedrop()
method?- a) Returns a copy of the DataFrame
- b) Modifies the original DataFrame without returning a copy
- c) Drops the column/row permanently from memory
- d) Creates a new DataFrame with the dropped rows/columns
How can you drop multiple columns at once from a DataFrame?
- a)
df.drop(columns=['col1', 'col2'])
- b)
df.drop(index=['col1', 'col2'])
- c)
df.drop(axis=1, labels=['col1', 'col2'])
- d)
df.drop(axis=0, labels=['col1', 'col2'])
- a)
Section B: Short Answer Questions
Dropping a Row: Given the following DataFrame, write the code to drop the row with the label '2'.
import pandas as pddata = {'A': [1, 2, 3], 'B': [4, 5, 6]}df = pd.DataFrame(data, index=[0, 1, 2])Dropping Multiple Rows: How would you drop multiple rows with labels '1' and '2' from a DataFrame? Write a code example.
Dropping Columns: Write a code snippet to drop the column
'B'
from the following DataFrame:import pandas as pddata = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}df = pd.DataFrame(data)Inplace Operation: Explain the difference between using
inplace=True
andinplace=False
when dropping rows or columns in a DataFrame.Drop with Conditions: Suppose you have a DataFrame with a column
'Age'
, and you want to drop all rows where the'Age'
is less than 20. Write the Python code to achieve this.
Section C: Practical Task
Create a DataFrame with columns
'EmployeeID'
,'Name'
,'Department'
, and'Salary'
containing at least 6 rows of data. Write the Python code to:- Drop the row of an employee with
EmployeeID: 103
. - Drop the
'Department'
column.
- Drop the row of an employee with
Using the DataFrame created in Task 1, drop all employees who belong to the
'HR'
department and store the result in a new DataFrame.
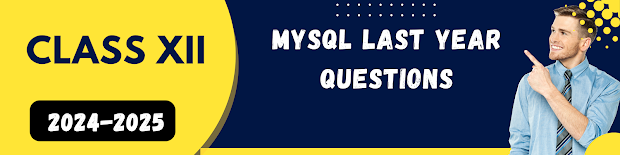.png)
0 Comments
Please do note create link post in comment section